Logical vs. Syntactical Errors in Code
What are Coding Errors?
Coding errors are problems or mistakes in code that prevent the program’s proper execution. These errors can take many forms and can prevent a program from either working as intended or from working at all. Coding errors are unavoidable in coding that programmers of all skill levels and experience levels will run into. Two of the most common types of errors that programmers experience are known as syntactical/syntax errors and logic errors.
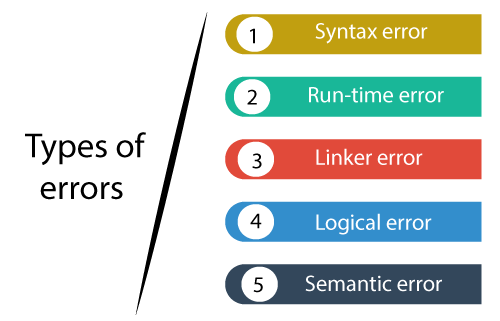
Syntactical Errors
Like any of the hundreds of different languages in use around the world, every coding language has its own set of rules that must be followed for its contents to make sense and have meaning. These rules are known as syntax. Unlike how human languages can still accurately convey their message despite grammatical flaws, coding languages have little to no room for errors. A syntax error such as a missing parenthesis or comma usually causes the program to fail to finish parsing or compile and halt in its tracks at the site of the error because it doesn’t know what to do due to the invalid instructions was handed.
Logic Errors
Logic errors, like syntax errors, also prevent a program from running in its intended fashion, but a program with a logic error is capable of running until completion. Generally, a program with a logic error creates an incorrect or undesirable output. This is because the code has proper instructions that follow syntax rules, but the programmer supplied it with instructions that do not accomplish the program’s intended purpose. Logical errors can be pretty difficult to detect because there isn’t a specific point of failure in the program that it can see itself. The programmer must scour the code to find the location of the error.

Examples of Coding Errors
Syntax Error Example in Python:

In this example, two variables named num_1 and num_2 are initialized with values of 23 and 4. A sum variable is then initialized with the sum of the previous two numbers, but one variable name is misspelled. This program wouldn’t run to completion because the num variable isn’t defined, and the program does not know what to do with the improper instructions it has been given, so it stops the program and sends to the console a NameError.
Syntax Error Example in JavaScript:

This example creates a function called multiplyNumbers that takes two parameters, num1 and num2, and returns their product. This function is then called to find the product of 3 and 44. However, when the program is run, the console gives an unexpected identifier syntax error. This error is caused by mistake made when declaring the function, as there is no opening curly brace to pair with the closing curly brace. JavaScript relies on those curly braces to contain all the steps that the function should execute when called, and since there isn’t an opening brace, it doesn’t know where to start and where to stop.
Logic Error Example in Python:

The program gets the user’s age by assigning the variable age to input in this example. The age is then evaluated in an if else statement to find if the age is above or below 18. Yet if this code is run, it outputs the opposite of what is intended. Inputting age as 12 results in an output of “You are an adult”, and inputting age as 45 outputs “You are not an adult”. This is due to a logic error on line 2. Instead of checking if the age variable is greater than or equal, it checks if age is less than or equal. By reversing the sign, the program can easily be fixed.
Logic Error Example in JavaScript:

The program above runs to completion because it is devoid of syntax errors; however, it does not output the correct average calculation. In this case, it outputs nine instead of the correct answer, 8. The logic error here is due to the order of mathematical operations, often called PEMDAS. When computing the variable’s value, “average” division happens before addition. Instead of dividing the sum of x and y by 2, it divided y by 2 and then adds x. This logic error can be solved by adding parenthesis around x and y: (x + y) / 2. This signifies that the sum of x and y should be divided.
Common Types of Syntactical and Logic Errors
Common Syntactical Errors
- Misspelling variable or function names.
- Missing or extra brackets/parenthesis/quotation marks.
- Using a variable before it has been declared.
- Incorrect usage of commas when separating different items in a list/array or dictionary/object.
- Using syntax rules for the wrong coding language
- Using = instead of == when checking if two things are equal.
- Doing math with a string that hasn’t been converted to a number.
Common Logic Errors
- Using incorrect operators.
- Using a greater than (>) sign instead of less than (<), or vice versa.
- Using the wrong variable for a calculation.
- Leaving a possibility of having an infinite loop that breaks the program.
- Using the same variable name more than once.
- Doing addition with strings consisting of numbers.
How to Avoid Programming Errors
Because errors are an unavoidable part of coding, finding and correcting them, also known as debugging, is a significant part of the building and upkeep of programs. The first step to avoiding errors starts during the program’s planning process. A programmer can pseudocode the program by identifying the inputs, processes, and outputs the program will be dealing with. Breaking the processes down into steps makes the code much easier to write and less prone to errors.
Pseudocode Example

Additionally, code must be tested as it is being written. Instead of writing a whole program and testing it at the end, test the program every few lines, only to find that it doesn’t work properly or at all. By ensuring that the program is initializing variables and doing the intended math properly as often as possible, programmers can avoid the headache of searching for and correcting multiple errors stacked onto each other. An extension of this is running programs through debuggers or linters occasionally to find syntactical mistakes and track down the root causes of logic errors.
Coding in the global scope, which makes variables available to other programs, can make a program conflict with other programs that share variable or function names, also called name collision. This can produce errors by causing an undesired function or variable to be used and can be avoided by coding in block scope and function scope or checking that there are no identical function or variable names.
Methods of Finding and Correcting Errors
Syntactical Errors
Because syntax errors cause a program to halt in its tracks, the cause of the error is usually returned to the console with a basic explanation of what caused the error.
Syntax errors are easy to solve because the information is handed to the programmer. If a program’s syntax error is a missing colon after a function declaration, the console will return “line 7 SyntaxError: expected ‘:’”. The program can be fixed by checking line 7 and adding a colon after the function declaration. Depending on the situation and type of error, the console entry may not be as specific in telling the cause of the error. However, the console will still return the line and sometimes the character position of the error. One must manually read the code to determine where the fault is in these situations.
Logical Errors
A program’s logical errors can be significantly harder to discover. Unlike syntax errors, the console will not tell where things went wrong because the code is working flawlessly from its perspective.
There are many ways of finding logical errors in code. Starting by analyzing the program’s output for clues that would lead to the part of the program that isn’t working as intended is usually the first step. For example, if a program does some arbitrary math with a few variables and finishes by printing a statement of “Your number is ‘NaN’” then one can identify that the value that is being printed isn’t recognized as a number by the program and check to see if the variables are numbers or were mistakenly turned into strings at some point. If the program prints “Your number is: 1” and the output is supposed to be a different number, then that information can be used to identify where the program’s math went wrong manually.
The best method for finding logical errors for smaller programs is to dry run the code. Dry running code is where a programmer manually runs through the program, keeping track of the values of variables along the way until they come across the location of the error or errors.
There also exist tools and software to assist programmers in tracking down logic errors. Many integrated development environments (IDEs) come packaged with a debugger that lets programmers walk through a program step-by-step and automates parts of dry running, such as automatically keeping track of variable values.

If all else fails, the rubber duck method is an option that can help programmers think through code differently. This debugging method is when a programmer talks to an inanimate object such as a rubber duck or teddy bear and explains in detail the problem they’re experiencing and the code’s overall objective, going line-by-line. Verbally describing the problem forces the programmer using this method to think about their program in greater detail, often eventually realizing their mistake.
Additional Types of Coding Errors
Runtime Error
A runtime error is a type of error where a successfully compiled, syntactically correct program suddenly terminates while running due to a mistake that is only detected when the program is run. Common types of runtime errors are dividing a number by 0, accessing non-existent indexes of arrays, and inputting non-number characters into an integer variable.
Resource Error
This type of error occurs when a computer’s allotment of resources has been exceeded and will not lend any more resources to a program. This can often be caused by running out of available random-access memory (RAM) or disk space. An infinite loop that creates a new array element every loop cycle would eventually create so many index locations that the computer has no more resources to develop more, causing a termination of the program.

Takeaway
In programming, errors are entirely unavoidable and happen to all skill and experience levels. Though there are many different types of errors that can be broken down into many subcategories, the two most common types are syntax and logical errors. These errors can range from simple typos of variable names to accidental infinite loops or math with words rather than numbers. While these errors become less common with experience, a good programmer should ideally know of several methods to deal with programs that have unknown mistakes in them. Whether utilizing the information, the console returns to them or learning how to use a debugger to track down a program’s logical errors efficiently.